JavaScript’s modern features—such as arrow functions, the spread operator, array and object methods, and new built-ins—enable developers to write elegant and concise solutions to common challenges. Tasks that once required dozens of lines of verbose code can often be reduced into a single, neat, and readable line. Below are the 10 best JavaScript one-liners that elegantly replace what used to be 50 (or more) lines of code.
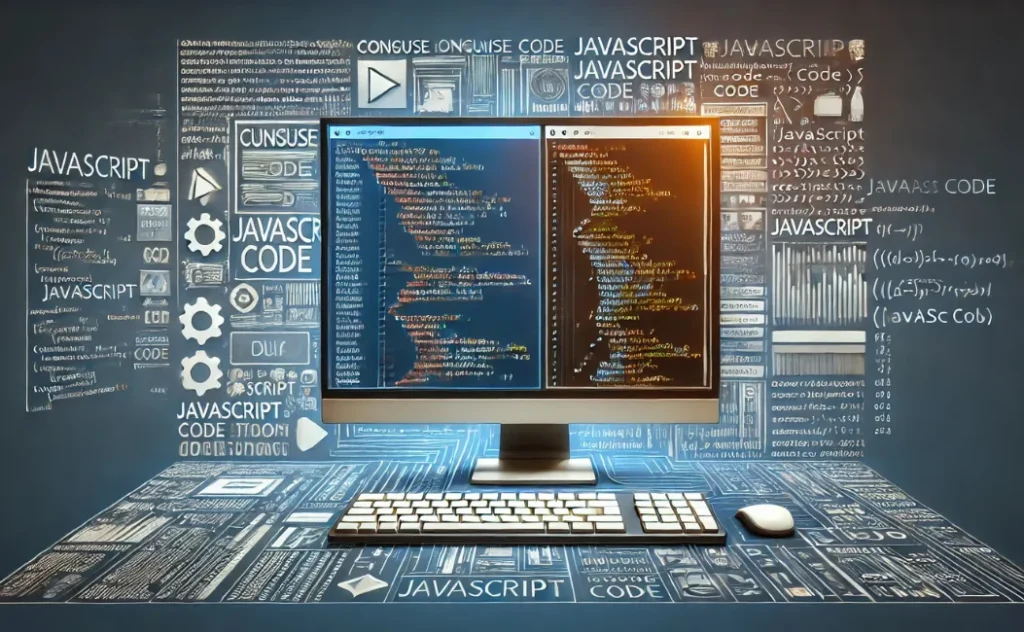
1. Remove Duplicates from an Array
Old Way: Manually loop and check if an element is in a temporary array.
One-Liner:
const uniqueArray = [...new Set(arr)];
What it Does:
Transforms an array into a Set
(which automatically removes duplicates) and then spreads it back into a new array.
2. Flatten a Deeply Nested Array
Old Way: Write a recursive function to check array depths.
One-Liner:
const flattenArray = arr => arr.flat(Infinity);
What it Does:
Uses flat(Infinity)
to fully flatten nested arrays, no matter how deep. Modern and incredibly neat.
3. Count the Frequency of Elements
Old Way: Use multiple loops or conditional statements to update counters.
One-Liner:
const frequency = arr.reduce((acc, x) => (acc[x] = (acc[x] || 0) + 1, acc), {});
What it Does:
Builds an object tallying how many times each element appears in the array.
4. Reverse a String
Old Way: Manually loop and concatenate characters in reverse order.
One-Liner:
const reversed = str.split('').reverse().join('');
What it Does:
Splits the string into an array of characters, reverses it, and then joins it back into a reversed string.
5. Check if a String is a Palindrome
Old Way: Loop over half the string and compare characters.
One-Liner:
const isPalindrome = str => str === str.split('').reverse().join('');
What it Does:
Compares the string with its reversed version. If they match, it’s a palindrome.
6. Merge Two Arrays
Old Way: Use loops or concat()
repeatedly.
One-Liner:
const merged = [...arr1, ...arr2];
What it Does:
Uses the spread operator to seamlessly merge two arrays into one.
7. Sort an Array of Objects by a Property
Old Way: Multiple lines of logic for custom sorting.
One-Liner:
const sorted = arr.sort((a, b) => a.prop - b.prop);
What it Does:
Uses sort()
with a simple comparison function to sort objects based on a specific property.
8. Shallow Clone an Object
Old Way: Manually copy each property.
One-Liner:
const clone = { ...obj };
What it Does:
The spread operator creates a shallow copy of the object easily.
9. Debounce a Function
Old Way: Write a full wrapper function managing timeouts.
One-Liner:
const debounce = (fn, ms) => { let t; return (...args) => (clearTimeout(t), t = setTimeout(() => fn(...args), ms)); };
What it Does:
Creates a debounced version of fn
that waits ms
milliseconds after the last call before executing, preventing repetitive work.
10. Local Storage: Object Storage and Retrieval
Old Way: Manually JSON.parse()
and JSON.stringify()
each time.
One-Liner:
const store = key => ({
get: () => JSON.parse(localStorage.getItem(key)),
set: data => localStorage.setItem(key, JSON.stringify(data)),
remove: () => localStorage.removeItem(key)
});
What it Does:
Wraps localStorage operations in a neat, JSON-aware API that saves and retrieves objects without repetitive boilerplate.
Conclusion
These 10 one-liners not only save keystrokes but also encapsulate common patterns in an expressive and maintainable form. Modern JavaScript’s powerful methods (like flat()
, fromEntries()
, Intl
, and Promise.allSettled()
) and concise syntax (spread operator, arrow functions) transform what used to be daunting tasks into elegant, self-documenting code.
Benefits:
- Improved Readability: Clear and concise expressions make the code easier to understand.
- Reduced Bugs: Fewer lines mean fewer opportunities for errors.
- Enhanced Maintainability: Simplified codebases are easier to manage and update.
Use these snippets as building blocks in your projects to enhance efficiency and focus on writing clear, logical code rather than managing boilerplate.